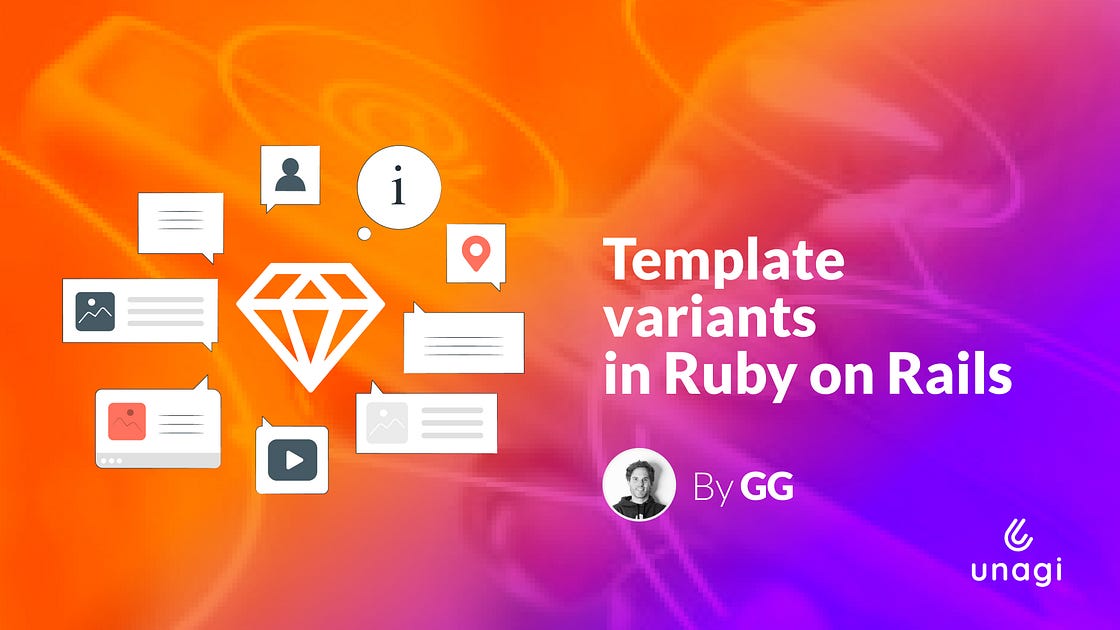
Were you aware of template variants in Ruby on Rails? It’s an option that the method render provides and can be used in our controllers to deal with customized layouts or views. Let’s see how it works with a couple of examples.
Some context
Typically, the controller’s render method is responsible for generating your application’s content for the browser. Various methods exist to customize the behavior of “render,” providing flexibility to render the default view for a Rails template, a designated template, a file, inline code, or opt for no rendering at all. You can even choose to render text, JSON, or XML.
Different views for admins and normal users in Ruby on Rails
Suppose we have one view for admins and a different one for the rest of the users (aka normal). We could easily differentiate the view to render by using variants
:
class ProductsController < ApplicationController
def index
request.variant = Current.user.admin? ? :admin : :user
end
end
- In the code above, every time an admin accesses our app it will render the view under the path
app/views/products/index.html+admin.erb
. - If it’s a normal user, the view path would be
app/views/products/index.html+user.erb
. - If none of those files is available, the path
app/views/products/index.html.erb
is used.
An ActionView::MissingTemplate
error is raised if none of the templates is available.
Different views for Mobile and Desktop in Ruby on Rails
Now, let’s suppose we have one view for mobile and one for desktop, and the views are too different to just be responsive. You can again use variants to specify which version to use by passing the :variants
option with a symbol or an array such as this:
# called in 'HomeController#index'
render variants: [:mobile, :desktop]
When using the option render variants: [:mobile, :desktop]
, Rails attempts to automatically determine which variant to use based on the User-Agent of the HTTP request — the user-agent is a header in the request that provides information about the user’s browser and OS —. This means that if the request comes from a mobile device, Rails will first look for the :mobile
variant, and if it’s for a desktop device, it will search for the :desktop
variant.
Going back to the set of variants above, Rails will look for the following set of templates in this order and use the first that exists:
app/views/home/index.html+mobile.erb
app/views/home/index.html+desktop.erb
app/views/home/index.html.erb
- If a template with the specified format doesn’t exist, an
ActionView::MissingTemplate
error is raised.
Conclusion
In conclusion, delving into the realm of template variants in Ruby on Rails unveils a powerful tool within the “render” method. This feature, embedded in controllers, empowers developers to craft tailored layouts and views, enhancing the adaptability of their applications. The flexibility of variants provides a nuanced approach.