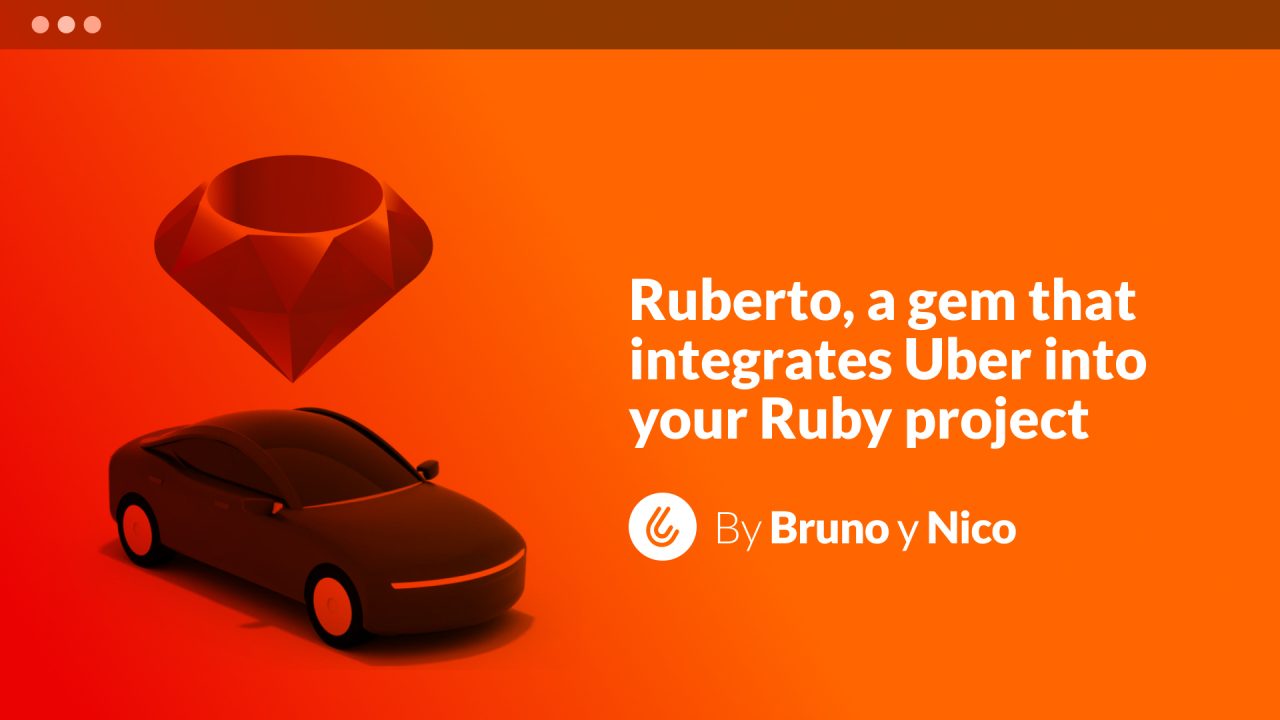
Ruberto is a Ruby gem that allows you to connect Uber’s API to any project. In this first release we focus on Uber Direct—the on-demand delivery service for businesses—although its modular design makes it possible to incorporate other Uber services in future versions. This open source project was born from the development of a Ruby on Rails application for one of our clients that needed to integrate home delivery quickly and efficiently. You can already install it from Rubygems and start using it in your project.
About Uber Direct
Uber Direct is Uber’s service tailored for shipping products, food, documents, or almost anything that needs transporting while designed for businesses to integrate delivery logistics into their systems. Unlike Uber Eats, which is aimed at the end consumer, Uber Direct enables companies to have a “virtual” fleet without the need to hire drivers or manage their own vehicles.
The Uber Direct API allows you to programmatically request drivers, track each shipment in real time, receive status notifications, and manage all the logistics associated with your deliveries. With this, any business can automate and optimize its delivery processes.
An API Wrapper for Your Deliveries
An API wrapper is, in simple terms, an abstraction layer that simplifies the use of an external API. Instead of dealing directly with HTTP endpoints, OAuth authentication, data conversion, and error handling, Ruberto encapsulates all this complexity in a straightforward interface following the key Ruby patterns.
Ruberto was created due to the needs of one of our clients, for whom we developed a Ruby on Rails application. The client is a food service company with a home delivery system. Although the Uber Direct API is fully functional, we realized we could save them time and code by creating a reusable abstraction. With Ruberto, connecting to Uber’s API becomes much simpler for anyone needing to develop a delivery service.
Integrating It Into Your Application
Integrating Ruberto is very straightforward. First, add the gem to your Gemfile:
gem 'ruberto'
If you use Rails, you can generate the configuration file with:
rails generate ruberto:init
This will create an initializer where you configure your credentials:
Ruberto.configure do |config|
config.customer_id = 'uber-customer-id'
config.client_id = 'uber-client-id'
config.client_secret = 'uber-client-secret'
end
Another key feature of Ruberto is its cache system for authentication tokens. By default, it uses a simple memory cache, but if you need a more robust solution, you can configure Ruberto to use alternatives such as Redis or the Rails cache. For example:
# To use Redis
Ruberto.cache = Redis.new
# To use the Rails cache
Ruberto.cache = Rails.cache
# Or to specify a file cache path (default)
Ruberto.configure do |config|
config.file_cache_path = 'tmp/ruberto_cache.yml'
end
This way, you won’t have to worry about manually handling expiring OAuth tokens.
Transforming Responses with the Magic of Ruberto::Object and Ruberto::Collection
One common challenge when working with external APIs is dealing with complex, nested data structures. To simplify this, Ruberto converts JSON responses from the Uber Direct API into Ruby objects with a clean and intuitive syntax.
Instead of navigating hashes like this:
response[:data][0][:dropoff][:contact][:first_name]
With Ruberto you can write it in a much more natural way:
deliveries.data.first.dropoff.contact.first_name
The magic happens in the Ruberto::Object class, which recursively transforms hashes into Ruby objects, and in Ruberto::Collection, which simplifies working with collections (including providing pagination link information). This makes your code more readable, safer, and less prone to errors due to typos in hash keys.
Suggestions and Contributions
Ruberto is an actively developed open source project, and we are very open to suggestions and contributions. Although it currently focuses on Uber Direct, its structure is designed to expand to other services in the future.
If you encounter any issues, have an idea to improve the gem, or have already implemented a solution, we ask that you:
1. Open an issue on our GitHub repository explaining the problem or suggestion.
2. Submit a pull request with your contribution.
3. Share your experience using Ruberto so we can continue improving it.
We’d also love to hear how you are using Ruberto in your projects. Real-world usage stories help us identify which features to prioritize for future releases.
If you have any questions about integrating Ruberto into your application, please start a discussion in the repository. We are committed to helping the community and ensuring you get the most out of this tool.
────────────────────────────
Feel free to try Ruber, and let us know how it works for you!