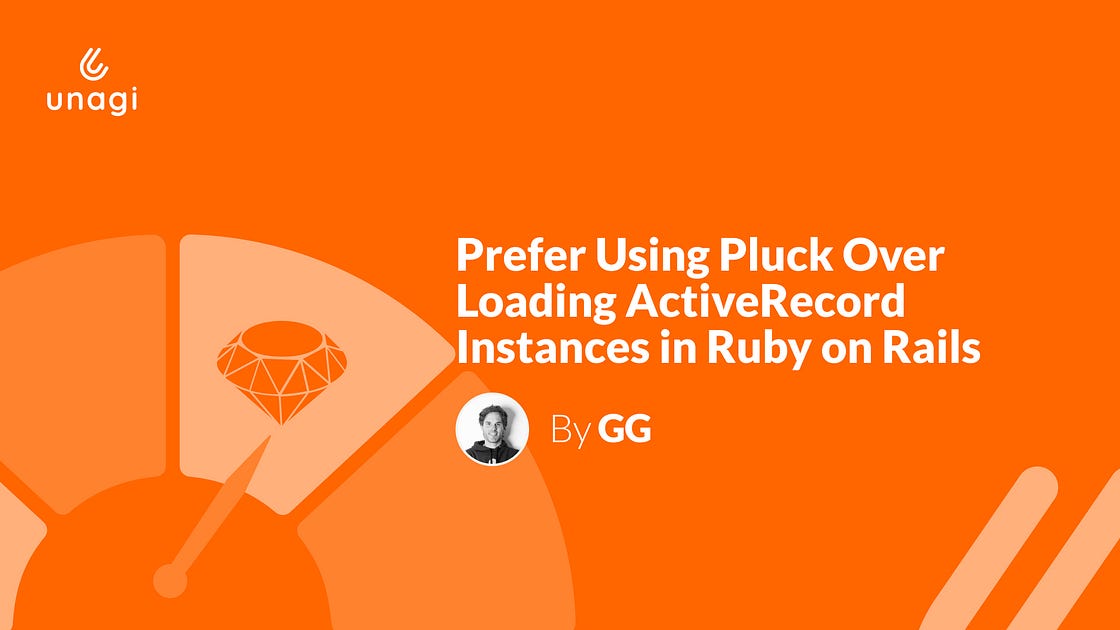
The pluck
method in Ruby on Rails provides a way to directly fetch specific columns from the database, returning the result as an array. This means that instead of retrieving full ActiveRecord instances with all their associated data and methods, you only get the values of the specified columns.
When you use pluck
to fetch data, it’s generally faster and consumes less memory compared to fetching entire ActiveRecord instances. This efficiency is particularly noticeable in scenarios where you only need certain attributes or when dealing with a large dataset, such as exporting data to CSV or text files with thousands of lines.
However, it’s important to be aware that using pluck
comes with a trade-off: since you’re not loading full ActiveRecord instances, you lose access to the model’s methods and associations. Therefore, it’s recommended to use pluck
when you specifically don’t need the functionality provided by your ActiveRecord model.
Let’s compare how pluck
could help us efficiently deal with a large text file. Let’s suppose we want to export a CSV file with a list of thousands of books in our database.
Without .pluck
CSV.generate do |csv|
Book.all.each { |book| csv << [book.isbn, book.title, book.author_name] }
end
With .pluck
CSV.generate do |csv|
Book.pluck(:isbn, :title, :author_name).each { |row| csv << row }
end
In terms of performance, the second code snippet is generally better than the first one.
- The
Book.pluck(:isbn, :title, :author_name)
query retrieves only the necessary columns from the database, the second approach fetches the entireBook
objects. Fetching only the required data reduces the amount of data transferred from the database to your application, resulting in faster execution. - The snippet with
Book.all
loads all book records as ActiveRecord objects. This involves object instantiation and consumes memory. On the other hand,pluck
directly retrieves an array of arrays from the database, avoiding the instantiation of full ActiveRecord objects. This is faster and consumes less memory. - Although both snippets use
CSV.generate
to create CSV data, the second snippet benefits from a more optimized CSV generation process by directly working with the array of arrays obtained from thepluck
query.
Conclusion
The pluck
method in Ruby on Rails allows you to only get the values of the specified columns, leading to improved performance. However, it’s essential to use it judiciously, considering that while it enhances efficiency, it comes at the cost of losing access to the model’s methods and associations.