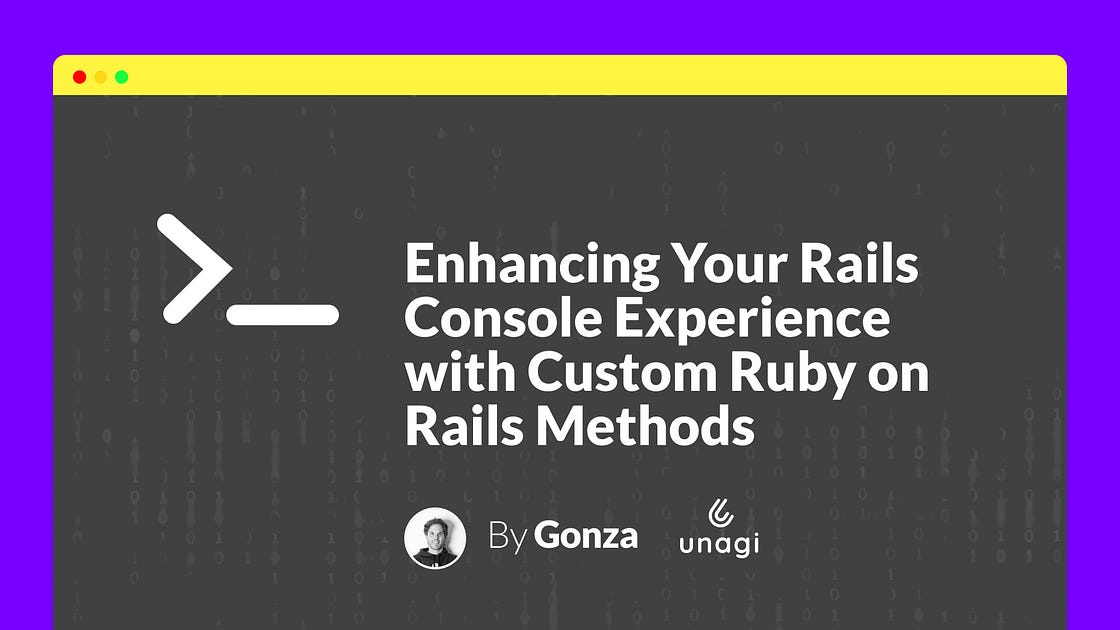
Did you know you can jazz up your Rails console with custom methods? It’s super handy for those everyday tasks, like logging in or measuring how long it takes to execute a code block.
To do that, you only need the module Rails::ConsoleMethods. Let’s see that in action.
User Login in Rails console
Add the following code in any initializer or environment file. For example, in config/environment/development.rb.
module Rails::ConsoleMethods
# This code sets the current user to the first user in the database.
def login
Current.user = User.first
end
end
By including this module in the environment file the login
method becomes available in the development Rails console. Now we can open the Rails console and type login
, which can be useful for testing or debugging scenarios where you need a logged-in user without manually going through the authentication process each time.
Measuring Code Block Execution Time in Rails Console
Same way, we can add a method named timer
to measure the time it takes to execute a block of code and then print the elapsed time in seconds.
module Rails::ConsoleMethods
# We capture the current time and store it in a variable. Then,
# we execute the code block that is passed to the timer method
# using the 'yield' keyword, and print the execution time.
def timer
start_time = Time.zone.now
result = yield
elapsed_time = Time.zone.now - start_time
puts "Execution time: #{elapsed_time.round(2)}s"
result
end
end
And here’s an example of how you might use this timer
method:
timer do
sleep(2) # or the code you want to measure
end
The console output would be something like:
Execution time: 2.0s