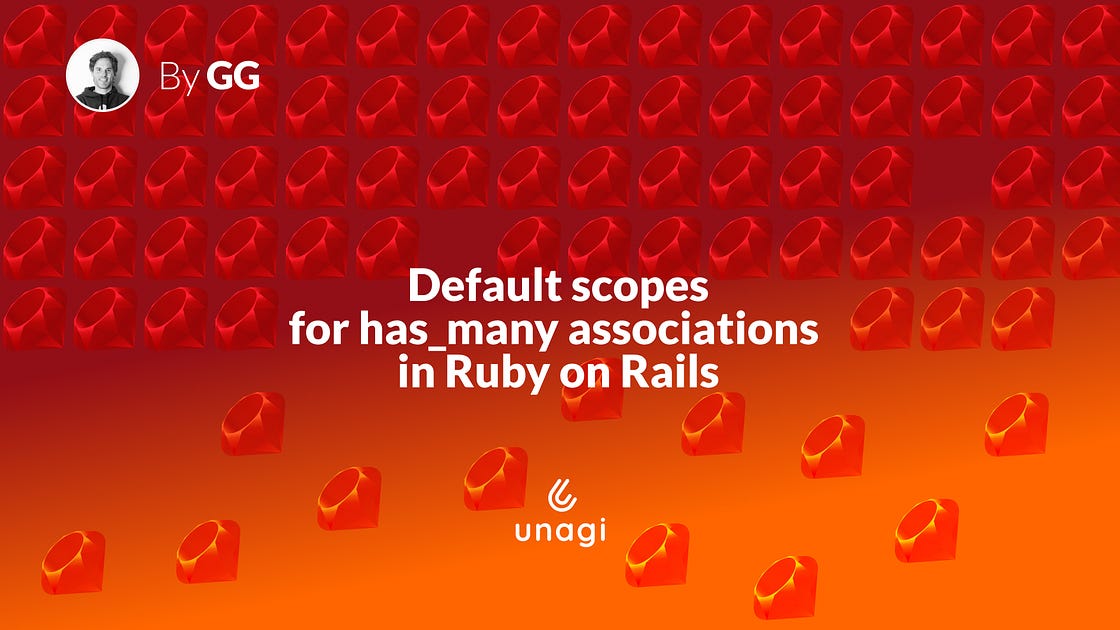
Ruby on Rails and Active Record offer a powerful feature that can enhance the efficiency of your code — default scopes for has_many
associations. However, with every great power comes great responsibility so be extremely cautious with default scopes. Otherwise, they will bite you back. If not used strategically, you might be leaving the door open for future problems.
But let’s see the whole picture…
Default Scopes in Ruby on Rails
In Rails’ world, managing associations is a common task. Imagine a scenario where a School
model has a has_many
association with students
. Without a default scope, retrieving and working with this association requires sorting or filtering each time it is referenced.
Code example 1: without default scope
# app/models/school.rb
class School < ApplicationRecord
has_many :students
end
# app/models/student.rb
class Student < ApplicationRecord
belongs_to :school
end
# Then, in the console, retrieving a collection of students from a school
# returns an unordered list.
School.first.students.map(&:name)
# => ["Pete", "Lucy", "Billy"]
In this example, every time you access the students
association, you’re left with an unordered list. Imagine having to repeat sorting or filtering logic across multiple parts of your application—inefficient and prone to errors.
Code example 2: without default scope
# app/models/school.rb
class School < ApplicationRecord
has_many :students, -> { order(:name) }
end
# In the console, retrieving a collection of students from a school
# The default scope automatically orders the elements
School.first.students.map(&:name)
# => ["Billy", "Lucy", "Pete"]
By setting a default scope for the students
association, we ensure that every query involving students automatically applies the specified order. This centralizes the sorting logic, providing cleaner, DRY (Don’t Repeat Yourself) code.
Unsorting for Flexibility: Unscope and Reorder
But what if you need to temporarily unsort or apply a different order? Rails provides solutions for that as well.
# Temporarily unsorting: Retrieve students in their natural order
School.first.students.unscope(:order).map(&:name)
# => ["Pete", "Lucy", "Billy"]
# Reorder with a different criterion
School.first.students.reorder(created_at: :desc).map(&:name)
# => ["Billy", "Lucy", "Pete"]
The unscope
method allows us to remove specific scopes temporarily, providing flexibility when the default order is not desired. On the other hand, reorder
allows us to apply a different ordering criterion on the fly.
While
unscope
is a powerful tool for overriding default scopes, its frequent use might indicate a structural issue in the approach to scoping. If you find yourself regularly needing to bypass the default scope, it might be a sign to consider an alternative strategy, such as using a named_scope.
Why Default Scopes Can Be a Future Problem
Default scopes can become problematic over time, especially when the application grows or when working on collaborative projects. Here are a few reasons why:
- Hidden Dependencies: Default scopes introduce implicit ordering or filtering, making it challenging to identify and manage dependencies. As the codebase evolves, these hidden dependencies can lead to unexpected behavior.
- Collaboration Challenges: In a collaborative environment, team members may not be aware of the default scopes applied, leading to unintentional conflicts and misunderstandings. It becomes crucial to communicate and document these scopes effectively.
- Flexibility Constraints: While default scopes provide convenience, they limit the flexibility to change ordering or filtering dynamically in different parts of the application. Over time, evolving requirements may necessitate dynamic adjustments, and default scopes can become a hindrance.
For example, consider a scenario where a new feature requires a different sorting criterion for the students association. If a default scope enforces a specific order across the application, accommodating this new requirement becomes challenging. Developers might resort to workarounds or frequent use of unscope
and reorder
, indicating potential structural issues. And this is just a minor new feature, you will probably have more complex use cases.
Conclusion
In conclusion, understanding default scopes for has_many
associations in Ruby on Rails might help you toward code optimization. But exercise discretion in their usage, as they may come back to haunt you. If the scope is more than just a basic sorting/ordering (like joins between tables), you might want to consider using named_scopes.
We will cover that in another article…